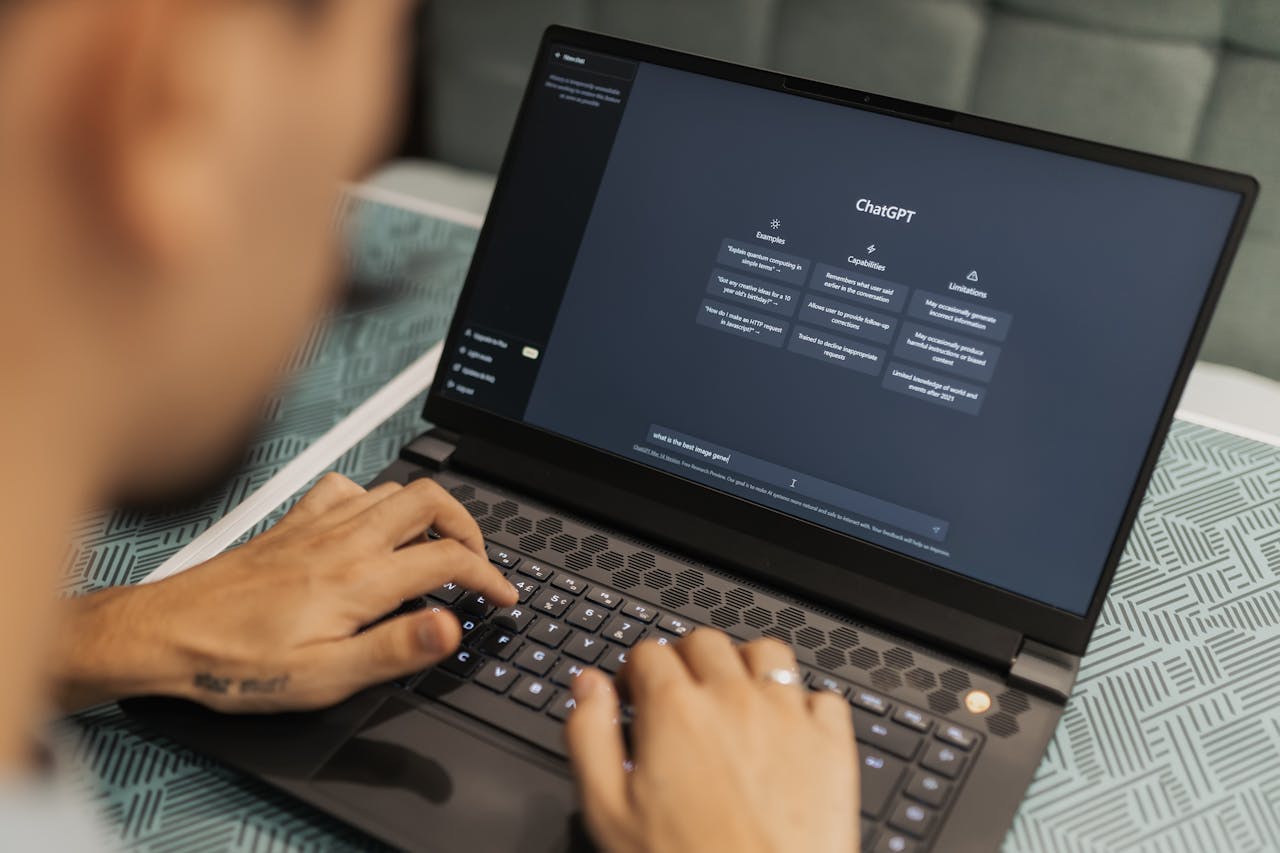
The OpenAI API has brought in innovative opportunities for developers and businesses, namely providing seamless access to advanced AI capabilities like ChatGPT for natural language processing, Codex for code assistance, and DALL·E for image generation. For cases like building more intelligent applications, automating workflows, or exploring generative AI, OpenAI’s versatile APIs help developers adopt these innovations most smoothly and efficiently.
Python is an excellent programming language for integrating OpenAI’s APIs. The core reasons are its simplicity, readable syntax, and vast ecosystem of libraries, satisfying all the requirements of AI-driven projects. OpenAI’s tools are designed to demonstrate seamless performance with Python, guaranteeing smooth integration, accelerated testing, and rapid deployment.
This guide walks you through the steps of how to integrate Open AI API with Python, taking you from initial setup and key acquisition to practical cases for text generation, code suggestions, and image creation. Besides, we’ll share optimization tips to manage your costs efficiently, maximize performance, deliver value and profit from the integration.
Table of Contents:
Prerequisites
Before diving into OpenAI API integration with Python, let’s make sure you have everything you need to get started. Follow the steps in the next section to set up your environment.
Basic Requirements
-
- Python installed. Confirm that Python is installed on your system. The OpenAI API is fully compatible with Python 3.7 or later, so using an up-to-date version will help eliminate compatibility issues.
- Python fundamentals. A basic knowledge of Python is highly recommended. You don’t need to be an expert, but familiarity with writing functions, importing libraries, and working with Python scripts will make the integration process smoother and more efficient.
Tools and Libraries Needed
Below, you can find all the necessary tools for seamless Open AI API integration.
Installations
Ensure you have installed the necessary Python libraries for seamless AI API integration. The openai
library is crucial for interacting with the API, while libraries like requests may come in use for handling additional HTTP requests if needed. These can be installed within a couple of clicks using pip commands, such as pip install openai
. Such libraries are the basis of seamless communication with the OpenAI API.
OpenAI API key
Next, you’ll need to obtain an API key from OpenAI. Start by creating an account on the OpenAI website and navigating to the API settings to generate your unique key. The aim of this key is to bridge your Python application and OpenAI’s servers, providing access to the API’s features. Please make sure to store it securely to prevent unauthorized access or misuse.
API documentation
Explore OpenAI’s comprehensive API documentation, which is absolutely packed with detailed guides, endpoint descriptions, and usage examples. This resource will serve as your support for troubleshooting, learning proven practices, and optimizing API integration. Bookmarking the documentation ensures it’s always within reach when you need a quick reference.
Setting Up the Environment
Before you can start working with Open AI API integration, set up your development environment properly. This process covers installing the necessary libraries, configuring your API key securely, and verifying that everything performs as expected. With the following steps, you can ensure a smooth integration process and eliminate any setup-related hiccups. Get your environment ready for Open AI API integration with Python.
Install the OpenAI Python Library
To begin, open your terminal or command prompt and install the OpenAI Python library using the following command:
pip install openai
This library simplifies interactions with the API by handling authentication, requests, and responses.
Set Up Your API Key
After installing the library, securely store your API key. It’s recommended to use environment variables for better security. For instance, on Linux or macOS, you can add this to your .bashrc
or .zshrc
:
export OPENAI_API_KEY='your-api-key-here'
In Python, retrieve it using:
import os
api_key = os.getenv('OPENAI_API_KEY')
Verify Installation
To verify everything is set up correctly, run this quick test in your Python environment:
import openai
import os
# Retrieve your API key from environment variables
openai.api_key = os.getenv('OPENAI_API_KEY')
# Test request: List available models
response = openai.Model.list()
print(response)
OpenAI API Basics
The OpenAI API is a versatile instrument created to integrate advanced AI capabilities into applications. Software engineers access state-of-the-art models within the API integration services to enable various functionalities, such as text generation, code assistance, and image creation, catering to different use cases.
Text Generation (ChatGPT)
One of the most versatile features of the OpenAI API is text generation powered by models like ChatGPT. It enables applications to generate coherent and contextually relevant text for a wide range of use cases, such as chatbots, content creation, summarization, and more. Covering use cases like AI assistant creation, story drafting, or automating customer support, Chat GPT API integration ensures natural language processing tools deliver accurate and engaging results. OpenAI offers multiple versions of ChatGPT, with the latest advancements like ChatGPT 4. It delivers enhanced precision, contextual comprehension, and response diversity compared to earlier versions like GPT-3.5.
Code Generation and Interpretation (Codex)
OpenAI offers Codex, a specialized model capable of interpreting, generating, and debugging code for software development tasks. It can transform natural language instructions into functional code snippets, optimizing the developers’ workflow. Codex streamlines performance by automating repetitive coding tasks, assisting with debugging, and even suggesting improvements to existing code. It is also compatible with a broad range of programming languages, including but not limited to the following ones:
-
- Python
- JavaScript
- Java
- C++
- C#
- Go
- Ruby
- PHP
- Shell scripting languages (e.g., Bash)
- HTML/CSS
- SQL
Image Generation (DALL·E)
For visual creativity, the OpenAI API features DALL·E, a model that generates unique images based on text prompts and given descriptions. This capability extends opportunities for designers, marketers, and creatives looking to create custom visuals, decreasing extensive manual engagement. The key objective of DALL·E is to simplify image creation while offering visually pleasing and highly customizable results.
Endpoints Overview
The API operates through specific endpoints, each tailored for different tasks. They are all important for structuring API requests effectively. OpenAI also provides detailed documentation outlining each endpoint’s parameters, expected outputs, and techniques. Having these insights in hand, developers can fully optimize the API to reflect the demands of their projects.
-
- Completions. For generating text based on prompts, ideal for chatbots or content creation.
- Edits. For modifying or refining existing text, such as rephrasing or correcting errors.
- Images. For generating unique images from textual descriptions using DALL·E.
Examples of OpenAI API Integration with Python
As we’ve already discussed, integrating OpenAI’s API into Python applications opens up a wide range of exciting perspectives to your software development direction, such as automating content creation, solving complex programming tasks, generating creative visuals, and more. We’ve compiled practical examples showcasing how to leverage OpenAI’s powerful tools, including ChatGPT for text generation, Codex for code assistance, and DALL·E for image creation. Each example demonstrates real-world applications that can elevate workflows for developers, content creators, and businesses.
Example 1: Text Generation with ChatGPT
ChatGPT excels at generating dynamic text-based responses. The following example demonstrates how to integrate Chat GPT API in Python within a simple script to create creative content like a short story. In this case, we use the gpt-3.5-turbo model to create human-like responses, perfect for storytelling, customer interaction, or content generation.
import openai
openai.api_key = "your-api-key"
response = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=[
{"role": "system", "content": "You are a creative storyteller."},
{"role": "user", "content": "Write a short story about a brave cat."}
],
max_tokens=100
)
print(response.choices[0].message["content"].strip())
Example 2: Summarizing Content
The API can simplify lengthy content into concise summaries. This caters to creating blog outlines, condensing reports, or extracting key points. The code delivers clear and actionable summaries by adjusting the prompt and token limits.
import openai
openai.api_key = "your-api-key"
prompt = "Summarize the following article: 'The rise of AI has transformed industries, including healthcare, finance, and transportation. Companies are using AI to automate tasks, gain insights, and improve decision-making.'"
response = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=[
{"role": "system", "content": "You summarize articles concisely."},
{"role": "user", "content": prompt}
],
max_tokens=50
)
print(response.choices[0].message["content"].strip())
Example 3: Code Assistance with Codex
Codex is a powerful tool for generating, debugging or optimizing code snippets. Below is an example of generating a Python function to calculate the Fibonacci sequence.
import openai
openai.api_key = "your-api-key"
prompt = "Write a Python function to calculate the Fibonacci sequence."
response = openai.Completion.create(
engine="code-davinci-002",
prompt=prompt,
max_tokens=50
)
print(response.choices[0].text.strip())
Example 4: Image Creation with DALL·E
DALL·E helps developers and designers generate outstanding visuals from simple text prompts. The output is a direct URL to the generated image, suitable for creative projects, visual marketing, or prototyping design ideas. Let’s see an example to create a futuristic cityscape image:
import openai
openai.api_key = "your-api-key"
response = openai.Image.create(
prompt="A futuristic cityscape at sunset",
n=1,
size="512x512"
)
print(response["data"][0]["url"])
Tips for Optimizing API Usage
Making the most of the OpenAI API requires thoughtful strategies to ensure efficiency, unlock new value streams, and ensure cost-effectiveness. Familiarize yourself with the tips below so you’ll be able to maximize the potential of the OpenAI API and seamlessly integrate it into your projects.
Efficient Prompt Engineering
Creating precise prompts is the primary aspect of achieving accurate and relevant outputs. A well-structured prompt provides context and clarity, minimizing iterative adjustments. For instance, instead of simply asking “Explain photosynthesis,” try adding context like, “Explain the process of photosynthesis to a 10-year-old student.” The specificity, as in the example, guides the API in producing responses that are aligned with your expectations, saving both time and tokens.
Cost Management
API costs can escalate quickly if not managed properly. To optimize usage, set token limits for your queries and choose the most cost-effective engine suited to your task. Put in practice, employ smaller models like text-curie-001
for straightforward tasks and reserve more robust models for complex requirements. Moreover, we highly recommend you track your usage through OpenAI’s dashboard to detect areas that lack efficiency.
Error Handling
Errors may occur when integrating APIs, especially if you’re new to the process of integrating APIs, but they’re manageable with a solid handling approach. The common issues technicians face are invalid API keys, exceeded rate limits, and improperly formatted requests. Use Python’s try-except
blocks to catch errors effectively and incorporate retries for transient issues. OpenAI’s error messages are typically descriptive for even more straightforward debugging.
Use Cases and Applications
The OpenAI API opens doors to a wide range of applications across many domains. Businesses, developers, and creatives have a robust, versatile instrument to innovate faster, more efficiently, and smarter. Applications ranging from automated customer service to advanced content generation and creative design tools can significantly elaborate with the OpenAI API integration.
Chatbots for Customer Service
OpenAI-powered chatbots can boost efficiency in handling customer requests. In fact, AI chatbots allow businesses to redirect 64% of agents’ focus toward resolving complex issues, according to Salesforce. These chatbots deliver instant responses, handle FAQs, and even escalate complex queries to human agents, enhancing customer satisfaction and boosting the service level while reducing support costs at the same time.
Content Generation for Marketing
The API automates content creation for marketing teams, from blog posts and social media captions to email campaigns. Streamlined workflows enable the production of high-quality material at scale. Marketing specialists can use the capabilities of OpenAI to maximize productivity, market analysis, and different types of research.
Automation of Repetitive Tasks
With the OpenAI API, businesses can delegate various repetitive and time-consuming tasks, such as summarizing lengthy reports, extracting key insights from unstructured data, and categorizing large datasets. This technology is beneficial in industries like finance, where it can streamline data analysis, or healthcare, serving as an assistant in processing patient records.
Creative Tools for Developers and Designers
Developers employ Codex to write and debug code and accelerate time-to-market. Designers, in turn, use DALL·E to create sophisticated visuals based on text provided by the tool. These solutions take the creative process to the next step, supporting rapid prototyping and cutting-edge problem-solving.
Advanced Concepts
Exploring advanced concepts allows you to experience the absolute capabilities of the OpenAI API, enabling specialized applications and large-scale implementations. Let’s discover how to fine-tune models to meet specific business needs and integrate the API with powerful tools and frameworks to achieve tailored, scalable, and efficient solutions.
Custom Fine-Tuning
For highly specialized use cases, fine-tuning OpenAI models can be customized. This process covers training the model on your proprietary data to elevate its performance for specific tasks, such as generating legal documents, analyzing niche datasets, or creating brand-aligned content. OpenAI provides tools to upload and train datasets, ensuring the model aligns with your business requirements while perfectly performing its core capabilities.
Scaling Applications with OpenAI API
When implementing large-scale applications, frameworks like Flask or Django help programmers deploy APIs as failure-resistant backend services. For instance, you can use Flask to create endpoints for text generation, making your AI solutions accessible to other services or users. Aside from that, techniques like adding caching mechanisms ensure faster responses, while load-balancing tools guarantee smooth scalability to handle increasing traffic with top-tier operational efficiency. It may slightly extend the time to integrate an API due to the additional training and testing phases, but the long-term benefits in precision and relevance make it well worth the investment.
Integration with Other Tools
Open AI API integration with platforms like Google Cloud, AWS, or Slack can enhance your functionality even more. In practice, you can integrate a Slack bot powered by ChatGPT to automate internal queries or combine OpenAI with AWS for real-time data analysis pipelines. Such integrations strengthen the value of the API by connecting it to broader ecosystems.
Common Challenges and How to Overcome Them
The OpenAI API can introduce some issues within its adoption, which is a common thing for any innovative solution or technology. Please take a look at the following potential API integration challenges so you know what to expect and how to achieve smoother implementation and better outcomes.
Rate Limits
A widely encountered hurdle is rate limiting, which restricts the number of API calls within a specific time frame. It can disrupt applications with high-volume requests. Optimize API usage by batching requests where possible, or consider upgrading your OpenAI subscription to higher limits to mitigate this. Implementing exponential backoff techniques in your code can also be an additional practice to handle rate-limit errors.
Model Performance
While OpenAI models are versatile, they may occasionally produce outputs that lack relevance or accuracy. This problem is often resolved with better prompt engineering. Refine your prompts by providing more precise instructions and relevant examples. If performance issues persist for specialized tasks, it’s suggested that the model be fine-tuned to provide more accurate and domain-specific outputs.
Debugging and Error Handling
Issues like invalid API keys, incorrect JSON formats, or connection errors can disrupt operations. To handle this, you can implement comprehensive debugging practices, such as logging error responses and testing endpoints with tools like Postman, speeding up resolution. Furthermore, incorporating try-except
blocks ensures that your application handles unexpected failures gracefully without crashing.
FAQ
In this guide, we’ve explored the full spectrum of Open AI API integration with Python. The adoption of Python’s rich ecosystem can help you seamlessly implement OpenAI’s powerful capabilities across diverse use cases, such as building chatbots, automating your domain-specific workflows, generating creative content, and more.
The OpenAI API’s advanced features enable businesses and developers to create scalable, bespoke, outstanding, sought-after solutions, providing everything needed.
Experiment with OpenAI’s tools, leverage resources like documentation and community forums and extend your software with the booming AI. Consider how AI can make your projects the following successful product with a vast range of innovative possibilities!
What is the cost of using OpenAI API?
The OpenAI API operates on a pay-as-you-go model, which basically means charging based on usage. The cost depends on the model (for instance, you are planning to integrate ChatGPT or Codex) and the number of tokens processed. OpenAI provides a pricing breakdown on its official pricing page. Don’t neglect to monitor your usage via OpenAI’s dashboard to avoid unexpected expenses.
Can I use OpenAI API for commercial projects?
Yes, OpenAI API can be used for commercial applications. The API is the best fit for business use within scenarios like creating chatbots for customer service, content generation tools, AI-driven applications, and more. Ensure compliance with OpenAI’s terms of service, particularly around the ethical use of AI and data privacy.
How do I secure my API key?
To prevent unauthorized access, store your API key securely in environment variables rather than hardcoding it into your scripts. Use tools like Python’s os
module to access these variables during runtime. Besides, we highly suggest rotating your API keys periodically and setting appropriate access restrictions within your OpenAI account to protect sensitive data.
If you have further questions or need more examples, OpenAI’s support resources and community forums are welcome to deepen your understanding and assist in handling specific challenges.