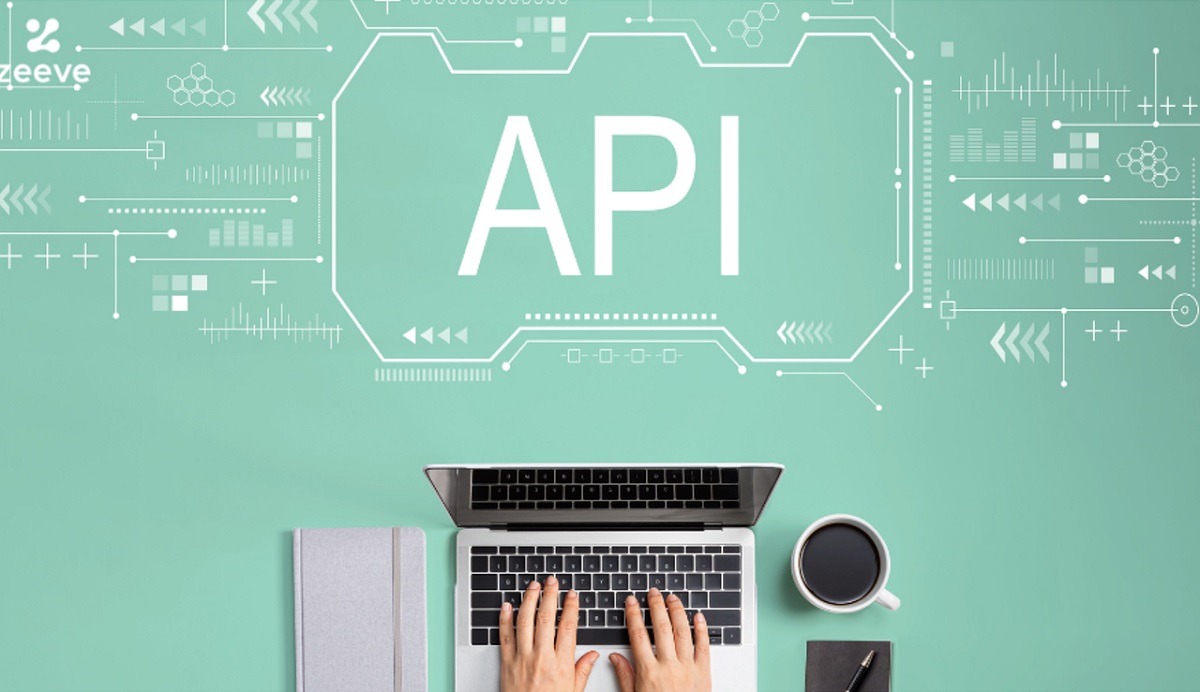
Application Programming Interfaces (APIs) play a critical part in establishing seamless communication and interaction between separate applications. They significantly streamline and enhance the way software is developed and deployed by providing standardized methods for applications to exchange data and functionality. Performing as bridges, APIs facilitate interoperability and enable developers to employ the strengths of multiple applications simultaneously.
Besides, APIs assist developers in creating modular, reusable code components that can be shared across projects and even among different teams. This promotes collaboration and accelerates development, as developers can focus on building specific features without having to learn the complicated peculiarities of the underlying systems. APIs also enhance security, as they allow controlled access to data and functionalities without exposing sensitive information.
The common examples of API integration are connecting an application to a database, integrating payment gateways, or linking different services in a microservices architecture. Within such activities, APIs streamline the development flow by encasing complex functionalities into more straightforward endpoints.
Among the broad spectrum of existing programming languages available, Python stands out as a relevant choice for API development. This is conditioned by its usability, clean syntax, versatility, and extensive ecosystem to choose from. With the power of the best Python framework for API development, such as FastAPI or Flask, and Django, technicians quickly prototype and implement APIs, as well as manage, and create documentation with ease.
Let’s discover how to develop an API in Python, best practices, security measures, and relevant tools to use.
Table of Contents:
Understanding APIs
An API serves as an indispensable intermediary that allows standalone software applications to connect, interact, and operate together effectively. It defines a set of rules, protocols, and tools that enable developers to access specific features or data from one application seamlessly and avoid underlying complexities.
Thus, they are responsible for the smooth integration between disparate systems, being a standardized bridge that enables applications to interact and transfer information, regardless of their architectures, languages, or platforms the solution is initially based on. This abstraction layer simplifies the development process, enhances collaboration among developers, and promotes the creation of modular, reusable code.
Why are APIs integral for software development? They bring significant benefits and features current software development landscape can’t do without:
- Interoperability. With APIs, different applications work in collaboration and are enabled to make use of each other’s strengths and capabilities. Put in practice, a mobile application may integrate APIs to connect with a server and retrieve data, even if the server is running on another technology stack.
- Modularity. APIs promote a modular approach to development, where complex functionalities are incorporated into self-contained units. This simplifies the development process as developers can focus on individual components without needing to manipulate and research the entire system.
- Code reusability. Developers reuse code and features across project, saving API development time and effort, as well as eliminating the need to recreate the same elements from scratch.
- Security. Controlled access points within an application’s data and functionalities are another benefit of APIs. It also enhances security by preventing unauthorized entry to sensitive information.
- Innovation. APIs enable developers to build upon existing platforms and services, achieving excellent, absolute software combining various technologies to create new and enhanced solutions
Unlock API Advantages
Discover the transformative power of APIs for your business growth.
In case these benefits are relevant for you, and you’re planning to create an API in the near future, it is important to consider API development cost. The core factors that form the final price are complexity, features, integrations, and the development team’s rates. A great value of Python is that it is open source, implying there are no licensing costs associated with using it for API development, ultimately leading to lower implementation and operational costs compared to other programming languages.
For an accurate estimate of API development services, we highly recommend you to apply to a credible tech vendor and request a precise price breakdown.
Before discussing how to build an API in Python, it is also important to mention that there are various types with their peculiarities as well as performance:
- Web APIs. These APIs, also known as HTTP APIs or RESTful APIs, enable communication over the internet using standard HTTP methods. Web APIs provide endpoints through which clients can request and manipulate data from a remote server. They are commonly used in web and mobile applications to fetch data from servers.
- Library APIs. This option provides pre-built functions, classes, and modules that developers can use within a single programming language or framework. They offer a set of tools to execute specific tasks eliminating the need to implement the functionality from scratch.
- Operating System APIs. Such APIs provide functions and services to interact with an operating system. They allow applications to perform tasks like file manipulation, memory allocation, and process management.
Designing Your API
Proper API design is crucial before implementation as it sets the basis for a successful and scalable system. A well-designed API ensures transparent communication among different software components and promotes long-term maintainability. One of the prevailing paradigms in API design is the principles of REST (Representational State Transfer),
REST API principles, founded on the concept of Representational State Transfer (REST), are a set of design guidelines that administer the development of web APIs. RESTful APIs provide a structured and standardized approach to building interfaces that facilitate the core purpose of APIs. The principles of REST have obtained widespread adoption due to their efficiency in ensuring high performance, scalability, and interoperability in up-to-date software development.
At its core, REST API principles is the idea of resources, which are entities that the API exposes and allows clients to interact with. Each resource is determined by a unique URL, delivering a consistent and intuitive way for clients to access particular assets, like data or functionality elements. Such a resource-centric strategy optimizes the API’s structure. These principles comprise:
- Statelessness. It implies that each request from a client to the server must contain all the information necessary to handle the request. The server does not reserve any details concerning the client’s afore-made requests so that each one is independent and self-contained. It enhances scalability, as servers can process requests in parallel without maintaining complex session states.
- Resource-based. RESTful APIs structure the application as a collection of resources determined by URLs (Uniform Resource Locators). These techniques can be manipulated using standard HTTP methods like GET, POST, PUT, and DELETE.
- Uniform interface. It’s a set of well-defined methods (HTTP verbs) that should be used to interact with resources. This consistency simplifies client-server interchanges and promotes a more transparent separation of concerns.
- Cacheability. Responses from the server can be marked as cacheable or non-cacheable. Therefore, it streamlines performance by reducing excessive data transfer.
- Client-server separation. The client and server are decoupled, which provides them with an opportunity to evolve independently. Such a separation enhances scalability and simplifies the development of different client applications.
- Layered system. An API can be organized using numerous layers, each responsible for a specific activity. It is important for flexibility and maintainability.
REST API principles are highly relevant in the context of software development. Primarily, they promote a clear separation of concerns between clients and servers, allowing for standalone development and elaboration of both sides. Besides, they enable easy integration with a broad range of platforms and programming languages, making it possible for diverse applications to communicate seamlessly. Adherence to REST principles also contributes to better performance and scalability, as the stateless nature and standardized methods facilitate efficient data exchange.
Another vital aspect of a successful API development with Python requires defining the integral elements we’ll list further. They are necessary for setting the way clients interact with your API, what actions they are able to accomplish, and how data is exchanged. In particular, they deliver a structured approach for designing Python APIs that follow the model of representing resources through URLs and utilizing standard HTTP methods for interaction.
Let’s begin a discussion on how to define these aspects in a way that ensures clarity, consistency, and usability within API development in Python.
Endpoints
They serve as the access points to the API, denoting particular functionalities or resources. When it comes to defining endpoints, it is crucial to strive for simplicity, transparency, and logical arrangement. For this, it’s critical to leverage descriptive and intuitive names that convey the purpose of the endpoint. You can also group related endpoints under consistent URL patterns to make navigation convenient. Versioning your endpoints will allow for future modifications not disrupting existing users. Last but not least, it’s critical to equip documentation for each endpoint, explaining its purpose, required parameters, and expected responses.
Methods
HTTP methods, such as GET, POST, PUT, and DELETE, define the actions that can be performed on endpoints. Your software engineers should manipulate methods in a manner that aligns with their intended purpose:
- GET for extraction of data
- POST for building new resources
- PUT for updating existing resources
- DELETE for removing resources
The chosen methods should be consistent with the nature of the endpoint and adhere to REST principles. Clearly document which methods are supported by each endpoint and the activities they execute to assist developers in comprehending how to interact with the API properly.
Payloads
It is the data transmitted between clients and servers in requests and responses. It’s noteworthy to design your payloads to be well-structured, consistent, and understandable. You may apply common formats like JSON or XML to ensure compatibility across different systems. For requests, define apparent rules for required and optional parameters, and validate incoming data to prevent errors. For responses, structure the data logically and include relevant metadata to aid client-side processing.
Error handling
Effective API design includes a comprehensive approach to error handling. This outlines standardized error response formats that furnish meaningful error codes, messages, and additional input to help in addressing issues.
Versioning
As your API extends, maintaining backward compatibility becomes vital. For this, you may consider implementing versioning strategies, such as including version numbers in the URL, headers, or through content negotiation. Thus, you can make changes in the API without disrupting the client software.
Handling Authentication and Authorization
Securing API with authentication and authorization mechanisms is of primary significance within the process of its creation. These measures are not only aimed at protecting sensitive data but also at ensuring the integrity of systems and services. Authentication validates the identity of users, while authorization governs their access to specific resources within the system.
Authentication methods offer various levels of security and flexibility within API development using Python.
- Token-based authentication implies handing out tokens, like JSON Web Tokens (JWTs), upon successful login. These tokens are then included in the headers of subsequent API requests, which allows the server to verify the user’s identity before processing the request.
- OAuth, on the other hand, is particularly useful for third-party access delegation. It allows users to give restricted access to their data without sharing their login details and elevating the security.
- Meanwhile, API keys, although straightforward, should be carefully employed, because they can easily be shared and misused.
Finally, the company can also establish role-based access control, assigning roles (such as admin, user, or guest) to users during registration. The assigned roles are stored in the database and are often comprised as part of the token payload upon successful authentication.
Endpoints and actions are configured to verify both the validity of the token and the associated role before approving access. This approach ensures that different user types possess special permissions based on their designated roles, effectively segmenting access privileges and enhancing across-the-board security.
Data Storage and Retrieval
Databases integrating APIs is a standard practice that enables the repository and retrieval of data in a structured and accurate strategy. Databases are in the role of storage hubs where information can be kept, organized, and further accessed through APIs, establishing seamless interaction between applications and data.
To integrate a database (like SQLite, MySQL, and PostgreSQL) with an API, there are particular steps to follow:
- Establishing a connection to the required database using appropriate libraries or frameworks. For instance, in Python, libraries like SQLAlchemy for PostgreSQL may be pretty relevant.
- Designing the database schema by defining tables, columns, and relationships that are suitable for the structure of your data.
After setting the database up, you may implement CRUD operations (Create, Read, Update, Delete) to interact with the data.
- Create (POST). To add new data, it’s necessary to send a POST request containing the required information to the API. With the use of the API, the request will be processed, inserting the data into the appropriate database table.
- Read (GET). Retrieving data engages sending a GET request to the API with specific parameters (e.g., an ID) to determine the desired data. The repository is queried by API and, returning the requested information in the response.
- Update (PUT/PATCH). To modify existing data, send a PUT or PATCH request along with the updated details to the API, which will in turn place the data in the database and update it accordingly.
- Delete (DELETE). Removing data occurs by sending a DELETE request with determining information to the API. The API will then remove the corresponding data from the database.
To illustrate CRUD operations in practice, imagine a plain task management software, where the API seamlessly integrates with a PostgreSQL database. Through the use of POST requests, you can generate new tasks, covering details such as title, description, and deadlines. By employing GET requests, tasks can be retrieved based on their unique ID or represented as a complete list. If there is a need for task modifications, PUT requests will come into relevance to update task specifics. Accordingly, DELETE requests would deliver a way to eradicate tasks entirely from the database.
Need Seamless API Integration?
Connect your systems and streamline your workflows with our expert API integration services.
Error Handling and Validation
Inalienable error handling is another point to take care of within API design, facilitating significantly to an elevated user experience and overall system solidity. These measures ensure that even in case something goes wrong with the performance, users receive clear and actionable feedback, rather than being met with confusing or cryptic error messages.
Implementing error responses and appropriate HTTP status codes is fundamental to effective error handling. When an error occurs, the API should respond with a structured error message, which might contain details like an error code, a user-friendly message, and possibly additional information for debugging. Subsequently, the HTTP status code in the response header should accurately reflect the essence of the error. For instance, every user has faced a 404 status code that indicates a resource was not found, whereas a 400 status code indicates a bad request.
Input validation is equally important in preventing false or vicious data from entering the API. Validating user inputs helps maintain data integrity, accuracy, and security. Techniques such as data type, length, and format validation help confirm that incoming data adhere to the necessary criteria.
- Data type validation confirms that the entered details match the expected data classification, preventing incompatible data from being processed.
- Length validation reviews the length of the entered data, preventing overly long or short data from the induction of issues.
- Format validation, especially for fields like email addresses or phone numbers guarantees that the data conforms to a specific pattern.
It is also wise to implement a scenario where a user submits a form to register for an application. Proper input validation will inspect if the entered email address sticks to a valid format, the password meets complexity requirements, and if other fields comprise precise and applicable data. If any validation fails, the API should respond with apparent error messages clarifying the matter, and assisting the user to fix their input.
API Documentation
Clear and comprehensive API documentation is essential for its successful implementation and the seamless integration of your services. With the use of proper documentation, tech professionals understand and employ your API effectively, while saving valuable time. Here’s why documentation matters:
- Software engineers’ onboarding. Documentation takes part as a guide for new developers, reducing the investigation time promoting quick adoption.
- Clarity and expectations. Transparent and in-depth documentation outlines what purposes the API executes, the way it performs, and the expected input/output, minimizing confusion.
- Optimized integration. Comprehensively documented endpoints, strategies, and parameters allow developers to integrate your API faster and decrease the number of errors.
- Troubleshooting and debugging. All-around instances and explanations assist in identifying and resolving challenges faster.
- Collaboration. Proper documentation facilitates collaboration among developers, both within your team as well as with external businesses.
To optimize API documentation in Python, consider using tools like Swagger and ReDoc. They streamline the flow of documenting APIs, simplifying the integration, and using the provided services.
Swagger
Swagger is an often employed framework that allows you to describe your API using the OpenAPI Specification (OAS). The OpenAPI Specification is a standardized approach to determine your API’s endpoints, request and response structures, authentication methods, and other. Swagger supplies tools to generate interactive documentation from your API’s OAS file. This documentation is called Swagger UI.
With Swagger, you get the following list of capabilities:
- Add notes to the API code with Swagger comments
- Generate an OAS file that thoroughly describes your API
- Use Swagger UI to build an interactive web-based documentation interface
- Allow developers to examine the API, and test endpoints
ReDoc
ReDoc is a tool designed to work in convergence with Swagger and the OpenAPI Specification mentioned earlier. While Swagger UI is feature-rich, ReDoc focuses on plainness, producing visually satisfying documentation from the API’s OAS file. ReDoc-generated documentation is responsive, easily navigated, and usable on various devices.
ReDoc is capable of the tasks mentioned below:
- Develop clean and appealing documentation from using OpenAPI Specification
- Customize the visual representation of the documentation to match your branding as needed
- Assure an intuitive and interactive interface for developers to explore endpoints and understand API leverage
In conjunction, Swagger and ReDoc offer a comprehensive solution for documenting your APIs. The beginning occurs by annotating the API code to create an OAS file using Swagger. Moving on, developers can employ either Swagger UI for feature-rich documentation or ReDoc for concise and straightforward one. These tools enhance the development flow by delivering valuable insights into your API’s functionality, and usage examples.
Testing Your API
API testing is essential to ensure its reliability, functionality, and robust performance. Comprehensive testing helps identify and fix issues early in development, leading to a more robust and trustworthy API operation.
Importance of Testing
Verifying that your API behaves as expected is the primary purpose of testing processes. It eliminates possible bugs, vulnerabilities in protection, and compatibility obstacles from reaching production. Properly tested APIs are more stable in their performance, providing better user experiences, and facilitating accelerated problem-solving within the development.
Types of Testing
Different types of testing for APIs serve specific purposes in ensuring the reliability, functionality, and performance of the API. Each type of testing targets specific aspects of the API’s behavior and interactions, contributing to a comprehensive testing strategy.
- Unit testing. This level of testing focuses on checking individual components or functions in isolation. Unit tests ensure that each part of your API performs as intended and can be executed rapidly.
- Integration testing. These tests verify that various components of your API work together seamlessly. It also ensures that different parts of the system can communicate and collaborate as required.
- End-to-end testing. Such tests simulate the complete user experience by examining the entire API workflow. It happens by validating that data flows correctly through the entire system, covering interactions with external services or databases.
How to write tests for your API endpoints in practice:
- Creating a user. Sending a valid POST request with complete user data. The goal is to ensure the API correctly processes the input, creates the user, and responds as required.
- Getting user information. Sending a GET request with a valid user ID and verifying that the API responds with the correct user information. This test also includes checking the cases where the user ID is not found, ensuring the API returns the expected error response.
- Handling errors. Testing various error scenarios, such as sending requests with incorrect parameters or invalid authentication tokens. Validating that the API generates the correct error responses, status codes, and error messages.
- Changing user data endpoint. Sending a PUT or PATCH request with modified user data and verifying that the API confirms the successful update. This also includes checking where the user ID is incorrect or the data provided is invalid, ensuring the API handles these situations appropriately.
- Testing authentication and authorization. Sending queries with valid and invalid authentication tokens to test secure endpoints. Verifying that unauthorized requests are met with the appropriate error responses.
- Testing data validation. Executing requests with various types of data to validate input validation mechanisms. Checking if invalid data formats are rejected and valid ones are processed correctly.
- Testing performance. Sending multiple requests concurrently to evaluate the API’s performance and response times. Monitoring memory usage to identify potential bottlenecks.
Deployment and Maintenance
When the testing phase is finished, the API is all set to release. Deploying and maintaining a Python API in a production environment involves several critical steps and ongoing practices to ensure its reliability and security:
- Setting up a production environment with necessary dependencies, databases, and configurations.
- Deploying your API code to the server leveraging version control systems.
- Configuring a web server to handle incoming API requests
- Deploying a WSGI server to serve your Python application
- Integrating to your production database and applying migrations if there’s such a necessity
- Enabling HTTPS for safe data exchange employing SSL certificates.
- Implementing load balancing to distribute traffic and provide sufficient availability
Maintaining and versioning your API comprises relevant practices to ensure its continued functionality and compatibility.
- Regular updates, such as bug fixes and feature enhancements, keep your API relevant and reliable.
- Implementing version numbers in your API endpoints helps maintain backward compatibility as modifications are executed
- Documentation is vital to guide both users and developers in comprehending your API’s services and proper utilization
- Automated testing addressed issues early on, boosting the whole quality of your API
- Version-controlled branches in your development process facilitate enhanced organization and collaboration.
- Having a well-defined rollback strategy equips you with handling unforeseen deployment challenges.
Lastly, monitoring, scaling, and addressing security concerns are critical points in maintaining a reliable and secure Python API. Monitoring ensures that the API functions optimally, helping to detect performance bottlenecks, error rates, and downtime much faster.
Scaling, another core aspect to consider, becomes essential as user demand raises, conditioning that the API handles higher traffic loads while maintaining responsiveness.
As safety is a primary focus within the API development with Python, security concerns should also be addressed. This may be completed with strategies like regular updates, encryption, and proper authentication. These measures safeguard sensitive data and protect the API from potential vulnerabilities, ensuring data integrity and user trust.
Employing these practices, you can guarantee the API’s reliability, efficiency, and data protection within its performance.
Our guide has uncovered the essential steps for API building in Python, using the relevant and up-to-date practices, catering to the security, scaling, and performance demands. Now equipped with a comprehensive understanding of each aspect of API development, you are aware of the approach a qualified IT vendor uses.
API creation requires strong technical expertise, careful planning, and experience in delivering IT services. Thus, the most efficient and optimized way to complete the API development successfully is by partnering with PLANEKS – your credible Python-based IT services vendor. Our comprehensive expertise tailored to your core objectives will help you introduce innovation, grow efficiency, partnership, establish a strong ecosystem, and bring in new value streams to your business.
Contact our certified team and employ our solid tech expertise to transform ideas into functional, secure, and compatible APIs.